Day 6 of TypeScript — As a Beginner
Things I’ve learned on day 6…
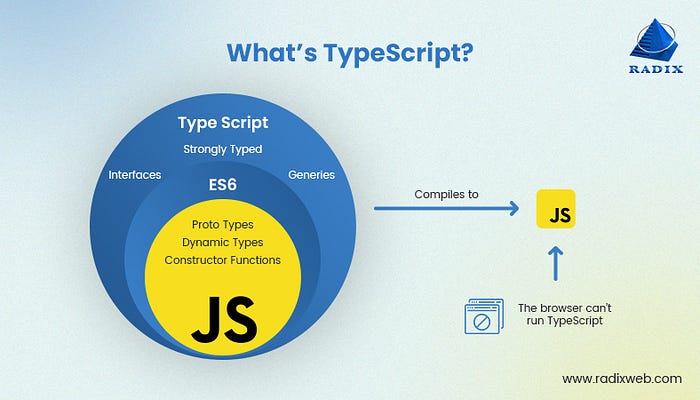
Things I’ve Learned today:
- Generics
- Classes
1. Generics
- Generics are the toolbox that enables us to create reusable components. A component should not always have to be React’s or TailwindCSS’s 😅.
- To understand this, let me show you an example which is in the TypeScript’s docs!
// Part one
function identity(arg: number): number { // 😐
return arg;
}
// Part Two
function identity(arg: any): any { // 😫
return arg;
}
- In part one, you can see that the
identity
function has an argumentarg
which is of typenumber
and returns it. - But what if we want that same function to be used with other types as well? You can of course use
any
type (as I’ve used it in the part two section of code) but that defeats the whole purpose of TypeScript! - Then what you can do to avoid this problem? Well, that is where the generics come into play! Let’s look at the example 👇
function genericFunction<Type>(argument: Type): Type {
return argument; // output: 69
}
genericFunction(69);
Code Explanation:
- Generics and the “any” type behave differently in terms of accepting and returning values.
- The “any” type allows for accepting and returning values of any type, without restrictions.
- Generics, on the other hand, enforce that the return type must be the same as the argument type.
- Generics provide type safety and maintain consistency between the argument and return types.
- The code example demonstrates a generic function that takes an argument of type
Type
and returns a value of the same type. - When calling the
genericFunction
with69
as the argument, it will return69
as the output, maintaining the same type. - Using the
any
type instead would allow accepting and returning values of any type, regardless of the specified argument and return types. - Generics offer a more structured approach to working with different types while ensuring type safety, compared to the more flexible nature of the “any” type.
Note:
It’s important to note the difference between generics and the
any
type. The “any” type allows accepting and returning values of any type, regardless of the specified argument and return types. For example, a function with the “any” type can receive astring
argument and return anumber
.However, generics behave differently. Generics restrict this behavior by enforcing that the return type must be the same as the type assigned to the argument. In other words, generics lock the return type to be consistent with the specified argument type.
Generics On Arrays
- Just like any other types in TS, Generics can also be used on Arrays!
Like so: 👇
/* --With Normal Functions-- */
// Generics on Arrays with normal function (TYPE: 1)
function arraysWithGenerics<Type>(array: Type[]) {
return array;
}
// Generics on Arrays with normal function (TYPE: 2)
function arraysWithGenerics<Type>(array: Array<Type>) {
return array;
}
/* --------------------------------------------------------------------- */
/* --With Arrow Functions-- */
// Generics on Arrays with arrow function (TYPE: 1)
const arraysWithGenerics = <Type>(array: Type[]) => {
return array;
};
// Generics on Arrays with arrow function (TYPE: 2)
const arraysWithGenerics = <Type>(array: Array<Type>) => {
return array;
};
console.log(arraysWithGenerics([1, 2, 3, 4, 5, 6]));
- There are two ways in which you can use arrays with Generics.
- The first one is by using
Type[]
and the other one is by usingArray<Type>
- Both of them work the exact same way! And all the above example functions work the exact same way! There’s no change in the behavior of the code at all. I just showed you the implementation of Arrays with Generics with both of these function types…😄
2. Classes
Quick Intro to Classes:
- Classes are templates for creating objects with similar properties and behaviors.
- They encapsulate data (properties) and functions (methods).
- Objects are created from classes using the
new
keyword. - Classes can have constructors to set initial property values.
- Inheritance allows classes to inherit properties and methods from other classes.
- Classes help organize and reuse code in an object-oriented manner.
class Animal {
animalType: string;
breed: string;
constructor(animal: string, breed: string) {
this.animalType = animal;
this.breed = breed;
}
displayOutput(): void {
console.log(
`Your pet animal is a ${this.animalType} and the breed is ${this.breed}`
);
}
}
const ShihTzu = new Animal("Dog", "Shih-Tzu");
ShihTzu.displayOutput();
The above code demonstrates the usage of a class called Animal
to create and display information about animal objects. The class has properties, a constructor, and a method for generating output. The code creates an instance of the Animal
class and calls the method to display specific details about the animal.
Code Explanation:
- It defines a class called
Animal
, which serves as a blueprint for creating animal objects. - The
Animal
class has two properties:animalType
, representing the type of the animal, andbreed
, representing the specific breed of the animal. - The class has a constructor, which is called when creating a new instance of the
Animal
class. - The constructor takes two parameters:
animal
(a string representing the animal type) andbreed
(a string representing the breed). - Inside the constructor, the parameter values are assigned to the corresponding class properties using the
this
keyword. - The
Animal
class also has a method calleddisplayOutput()
, which logs a formatted message to the console, displaying the animal type and breed. - An instance of the
Animal
class is created using thenew
keyword, with the animal type set to "Dog" and the breed set to "Shih-Tzu". This instance is assigned to the variableShihTzu
. - Finally, the
displayOutput()
method is called on theShihTzu
instance, which prints the message to the console, indicating that the pet animal is a Dog and the breed is Shih-Tzu.
These are the things that I’ve learned today that is Day 6!
Hope you got some useful information from this blog. If you did make sure to follow me as we are nearing 10 followers!🔥🎉
Goodbye…for now!👋😁
Here are my socials if you’re interested in connecting with me:
Yeah. That’s it.😅😂